In this article, I will explain how can you load partial view using jquery ajax inside main view and show data without redirecting to another page using Bootstrap pop-up modal in ASP.NET MVC
Step 1: Create a project in your Visual Studio(2017 in my example), by opening Visual Studio and clicking "File"-> "New"-> "Project".
Select MVC template to generate basic HomeController
and other details.
Step 2: Now we need to connect our project with database, so get your database from SQL server and connect it with your MVC project, here I am using my local database "Students", with table "Student_table" here is the snapshot of database, with its current dummy data
You can create database with dummy database with Demo table and Data using the below scripts (using SQL server 2012 or above)
USE [master]
GO
/****** Object: Database [Students] Script Date: 2/12/2018 9:06:00 PM ******/
CREATE DATABASE [Students]
CONTAINMENT = NONE
ON PRIMARY
( NAME = N'Students', FILENAME = N'c:\Program Files\Microsoft SQL Server\MSSQL11.MSSQLSERVER\MSSQL\DATA\Students.mdf' , SIZE = 3072KB , MAXSIZE = UNLIMITED, FILEGROWTH = 1024KB )
LOG ON
( NAME = N'Students_log', FILENAME = N'c:\Program Files\Microsoft SQL Server\MSSQL11.MSSQLSERVER\MSSQL\DATA\Students_log.ldf' , SIZE = 1024KB , MAXSIZE = 2048GB , FILEGROWTH = 10%)
GO
ALTER DATABASE [Students] SET COMPATIBILITY_LEVEL = 110
GO
IF (1 = FULLTEXTSERVICEPROPERTY('IsFullTextInstalled'))
begin
EXEC [Students].[dbo].[sp_fulltext_database] @action = 'enable'
end
GO
ALTER DATABASE [Students] SET ANSI_NULL_DEFAULT OFF
GO
ALTER DATABASE [Students] SET ANSI_NULLS OFF
GO
ALTER DATABASE [Students] SET ANSI_PADDING OFF
GO
ALTER DATABASE [Students] SET ANSI_WARNINGS OFF
GO
ALTER DATABASE [Students] SET ARITHABORT OFF
GO
ALTER DATABASE [Students] SET AUTO_CLOSE OFF
GO
ALTER DATABASE [Students] SET AUTO_CREATE_STATISTICS ON
GO
ALTER DATABASE [Students] SET AUTO_SHRINK OFF
GO
ALTER DATABASE [Students] SET AUTO_UPDATE_STATISTICS ON
GO
ALTER DATABASE [Students] SET CURSOR_CLOSE_ON_COMMIT OFF
GO
ALTER DATABASE [Students] SET CURSOR_DEFAULT GLOBAL
GO
ALTER DATABASE [Students] SET CONCAT_NULL_YIELDS_NULL OFF
GO
ALTER DATABASE [Students] SET NUMERIC_ROUNDABORT OFF
GO
ALTER DATABASE [Students] SET QUOTED_IDENTIFIER OFF
GO
ALTER DATABASE [Students] SET RECURSIVE_TRIGGERS OFF
GO
ALTER DATABASE [Students] SET DISABLE_BROKER
GO
ALTER DATABASE [Students] SET AUTO_UPDATE_STATISTICS_ASYNC OFF
GO
ALTER DATABASE [Students] SET DATE_CORRELATION_OPTIMIZATION OFF
GO
ALTER DATABASE [Students] SET TRUSTWORTHY OFF
GO
ALTER DATABASE [Students] SET ALLOW_SNAPSHOT_ISOLATION OFF
GO
ALTER DATABASE [Students] SET PARAMETERIZATION SIMPLE
GO
ALTER DATABASE [Students] SET READ_COMMITTED_SNAPSHOT OFF
GO
ALTER DATABASE [Students] SET HONOR_BROKER_PRIORITY OFF
GO
ALTER DATABASE [Students] SET RECOVERY SIMPLE
GO
ALTER DATABASE [Students] SET MULTI_USER
GO
ALTER DATABASE [Students] SET PAGE_VERIFY CHECKSUM
GO
ALTER DATABASE [Students] SET DB_CHAINING OFF
GO
ALTER DATABASE [Students] SET FILESTREAM( NON_TRANSACTED_ACCESS = OFF )
GO
ALTER DATABASE [Students] SET TARGET_RECOVERY_TIME = 0 SECONDS
GO
USE [Students]
GO
/****** Object: Table [dbo].[StudentTable] Script Date: 2/12/2018 9:06:00 PM ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[StudentTable](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Name] [varchar](50) NULL,
[StudentClass] [varchar](50) NULL,
[Subjects] [varchar](50) NULL,
CONSTRAINT [PK_StudentTable] PRIMARY KEY CLUSTERED
(
[Id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
SET ANSI_PADDING OFF
GO
SET IDENTITY_INSERT [dbo].[StudentTable] ON
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass], [Subjects]) VALUES (1, N'Ramesh', N'XI', N'Arts')
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass], [Subjects]) VALUES (2, N'Suersh', N'XI', N'PCM')
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass], [Subjects]) VALUES (3, N'Pretty', N'XII', N'PCM')
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass], [Subjects]) VALUES (5, N'Testing new entry', N'XII', N'Commerce')
SET IDENTITY_INSERT [dbo].[StudentTable] OFF
USE [master]
GO
ALTER DATABASE [Students] SET READ_WRITE
GO
Step 3: Let's connect to the database. so create Entity framework(ADO.NET) file to connect the project with the database, you would have to add Entity Data Model to your project by right-clicking the "Model" folder in your Solution Explorer and then click on Add and then New Item option of the Context Menu.
From the Add New Item window, select ADO.NET Entity Data Model and set its Name as "StudentModal" and then click Add.
Then the Entity Data Model Wizard will open up where you need to select EF Designer database option.
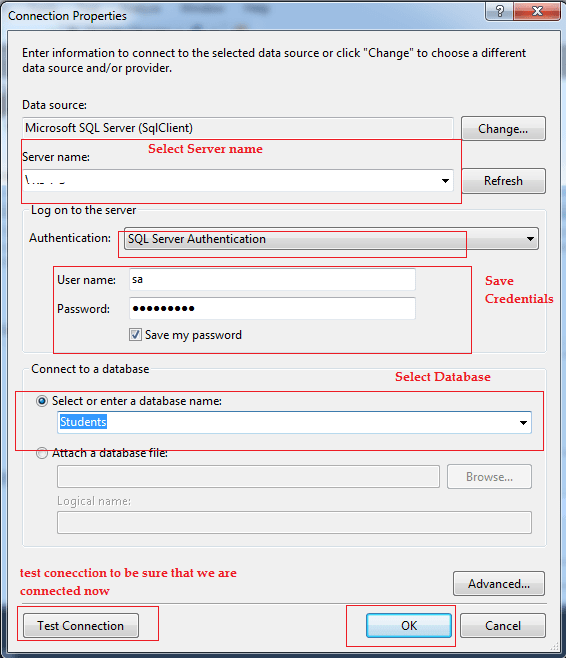
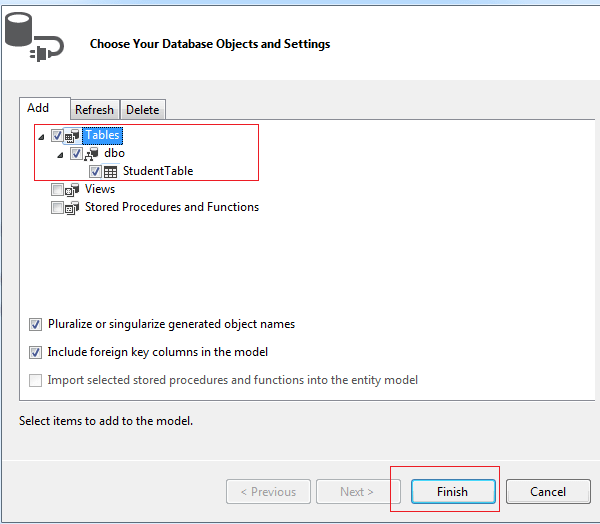
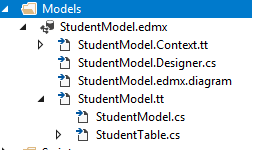
HomeController
Index action method, fetch all the details to show data in Index view using the code belowpublic ActionResult Index()
{
StudentsEntities entities = new StudentsEntities();
return View(from students in entities.StudentTables.Take(10)
select students);
}
[HttpPost]
public ActionResult Details(int studentId)
{
StudentsEntities entities = new StudentsEntities();
return PartialView("Details", entities.StudentTables.Find(studentId));
}
In the above code, using Index ActionMethod we are fetching all the list of students, while using Details ActionMethod, we are selecting specific student and passing it to PartialView
Now in you Index view, use the code below to show all students
@model IEnumerable<LoadPartialViewUsingjQueryAjax.Models.StudentTable>
@{
ViewBag.Title = "Home Page";
}
<h4>Students</h4>
<hr />
<table class="table table-bordered" id="StudentGrid">
<tr>
<th>Id</th>
<th>Student Name</th>
<th>Class</th>
<th>Subject</th>
<th></th>
</tr>
@foreach (var student in Model)
{
<tr>
<td>@student.Id</td>
<td>@student.Name</td>
<td>@student.StudentClass</td>
<td>@student.Subjects</td>
<td><a class="btn btn-primary details" href="javascript:void(0)" >View</a></td>
</tr>
}
</table>
<!--Bootstrap Pop Up modal-->
<div id="myModal" class="modal fade" role="dialog">
<div class="modal-dialog">
<!-- Modal content-->
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Student details</h4>
</div>
<div class="modal-body" id="ModalBody">
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<!--End of Bootstrap Pop-Up modal-->
As discussed earlier we will be showing PartialView data in Bootstrap Pop-up modal, so I have it's HTML code also but wit empty modal-body.
Now to load the Partial View using jQuery ajax & show it in modal pop-up, here is the jQuery code for it
$(function () {
$("#StudentGrid .details").click(function () {
//get student id of row clicked
var studentId = $(this).closest("tr").find("td").eq(0).html();
$.ajax({
type: "POST", //Method type
url: "/Home/Details", //url to load partial view
data: '{studentId: "' + studentId + '" }', //send student id
contentType: "application/json; charset=utf-8",
dataType: "html",
success: function (response) {
$('#ModalBody').html(response);
$('#myModal').modal('show');
},
failure: function (response) {
alert(response.responseText);
},
error: function (response) {
alert(response.responseText);
}
});
});
});
I have explained few lines of javascript code using comments, so complete Index View code will be as below:
@model IEnumerable<LoadPartialViewUsingjQueryAjax.Models.StudentTable>
@{
ViewBag.Title = "Home Page";
}
<h4>Students</h4>
<hr />
<table class="table table-bordered" id="StudentGrid">
<tr>
<th>Id</th>
<th>Student Name</th>
<th>Class</th>
<th>Subject</th>
<th></th>
</tr>
@foreach (var student in Model)
{
<tr>
<td>@student.Id</td>
<td>@student.Name</td>
<td>@student.StudentClass</td>
<td>@student.Subjects</td>
<td><a class="btn btn-primary details" href="javascript:void(0)" >View</a></td>
</tr>
}
</table>
<!--Bootstrap Pop Up modal-->
<div id="myModal" class="modal fade" role="dialog">
<div class="modal-dialog">
<!-- Modal content-->
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Student details</h4>
</div>
<div class="modal-body" id="ModalBody">
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
<!--End of Bootstrap Pop-Up modal-->
@section scripts{
<script type="text/javascript">
$(function () {
$("#StudentGrid .details").click(function () {
//get student id of row clicked
var studentId = $(this).closest("tr").find("td").eq(0).html();
$.ajax({
type: "POST", //Method type
url: "/Home/Details", //url to load partial view
data: '{studentId: "' + studentId + '" }', //send student id
contentType: "application/json; charset=utf-8",
dataType: "html",
success: function (response) {
$('#ModalBody').html(response);
$('#myModal').modal('show');
},
failure: function (response) {
alert(response.responseText);
},
error: function (response) {
alert(response.responseText);
}
});
});
});
</script>
}
Step 5: Now in this step, we will create Partial View for Details ActionMethod, so go to HomeController, right click on Details View and click on "Add View"
In the generated partial View, we will Show Student name, Class, Subject using HTML
@model LoadPartialViewUsingjQueryAjax.Models.StudentTable
<div class="row">
<div class="col-lg-2"><label>Name</label></div>
<div class="col-lg-10">@Model.Name</div>
</div>
<div class="row">
<div class="col-lg-2"><label>Class</label></div>
<div class="col-lg-10">@Model.StudentClass</div>
</div>
<div class="row">
<div class="col-lg-2"><label>Subjects</label></div>
<div class="col-lg-10">@Model.Subjects</div>
</div>
Step 6: This is final step, build your project and run it in browser, you will get the below output
If you will click on "View" button of any row, jQuery code will call Details ActionMethod using AJAX which returns us the HTML of Partial View, on success of Ajax, we are appending that HTML string in the Modal Pop-up body and opening/showing it.
That's it, we have successfully loaded the partial view in Bootstrap pop-up modal using jQuery Ajax.
Here is the Google drive link to the complete Source code available to download https://drive.google.com/file/d/1KJpAV8E21oeo_2N1E8hVQd3epz8C3iVY/view?usp=sharing
Note: Above link doesn't include database file, so you can copy script from the article's Step 2 or modify code according to your database tables.
Feel free to ask questions, related to this article in questions section, by linking it or comment below in comment's section.