This article will help you perform Create, read, update, and delete (CRUD) operations in asp.net MVC using jqGrid, jqGrid plugin is alternative to jQuery datatable and can be used with MVC projects to show a data in table and perform basics CRUD operations, you can check two-part jQuery Datatable CRUD operations step by step using Web-API also
jQGrid Introduction
jqGrid is an AJAX-enabled grid plugin for the javascript which provides complete solutions for representing & manipulating tabular data on the web. Because it is a jQuery plugin it will work only on client side not on server side but for binding the data which will be on server side it can be loaded dynamically using ajax call. This is a free and open source library, which is distributed under MIT license. Actually, this plugin mainly targets the PHP as the backend. But, it can be integrated with any server-side technology, including PHP, ASP, Java Servlets, JSP, ColdFusion, and Perl.
Important Features
- AJAX enabled
- Accepts XML/JSON/local array data sources
- Import and Export Data
- Support of 20 languages of
- Supports paging, sorting, and filtering
- Supports inline editing
- Sub-grid & grid details
- Grouping
- UI datepicker integration
Implementing CRUD using jqGrid in ASP.NET MVC
Step 1: Open your Visual Studio(here using 2017 community version) and create a new project (File -> New -> Project)
Step 2: Select Asp.net Web application and give a name (jqGridMVC)
Step 3: Select MVC template
Step 4: Right-click on your project(In Solution Explorer pane) -->Manage Nuget packages-->In "Nuget package manager window" select the "Browse" tab, then search for "jquery.Jqgrid" , Select and click install.
Step 5: Get database in SQL server, here i am using my local database "Students", with table "StudentTable" here is the snapshot of database, with its current dummy data
You can create database with dummy database using the below scripts (using SQL server 2012 or above)
USE [master]
GO
CREATE DATABASE [Students]
CONTAINMENT = NONE
ON PRIMARY
( NAME = N'Students', FILENAME = N'c:\Program Files\Microsoft SQL Server\MSSQL11.MSSQLSERVER\MSSQL\DATA\Students.mdf' , SIZE = 3072KB , MAXSIZE = UNLIMITED, FILEGROWTH = 1024KB )
LOG ON
( NAME = N'Students_log', FILENAME = N'c:\Program Files\Microsoft SQL Server\MSSQL11.MSSQLSERVER\MSSQL\DATA\Students_log.ldf' , SIZE = 1024KB , MAXSIZE = 2048GB , FILEGROWTH = 10%)
GO
ALTER DATABASE [Students] SET COMPATIBILITY_LEVEL = 110
GO
IF (1 = FULLTEXTSERVICEPROPERTY('IsFullTextInstalled'))
begin
EXEC [Students].[dbo].[sp_fulltext_database] @action = 'enable'
end
GO
ALTER DATABASE [Students] SET ANSI_NULL_DEFAULT OFF
GO
ALTER DATABASE [Students] SET ANSI_NULLS OFF
GO
ALTER DATABASE [Students] SET ANSI_PADDING OFF
GO
ALTER DATABASE [Students] SET ANSI_WARNINGS OFF
GO
ALTER DATABASE [Students] SET ARITHABORT OFF
GO
ALTER DATABASE [Students] SET AUTO_CLOSE OFF
GO
ALTER DATABASE [Students] SET AUTO_CREATE_STATISTICS ON
GO
ALTER DATABASE [Students] SET AUTO_SHRINK OFF
GO
ALTER DATABASE [Students] SET AUTO_UPDATE_STATISTICS ON
GO
ALTER DATABASE [Students] SET CURSOR_CLOSE_ON_COMMIT OFF
GO
ALTER DATABASE [Students] SET CURSOR_DEFAULT GLOBAL
GO
ALTER DATABASE [Students] SET CONCAT_NULL_YIELDS_NULL OFF
GO
ALTER DATABASE [Students] SET NUMERIC_ROUNDABORT OFF
GO
ALTER DATABASE [Students] SET QUOTED_IDENTIFIER OFF
GO
ALTER DATABASE [Students] SET RECURSIVE_TRIGGERS OFF
GO
ALTER DATABASE [Students] SET DISABLE_BROKER
GO
ALTER DATABASE [Students] SET AUTO_UPDATE_STATISTICS_ASYNC OFF
GO
ALTER DATABASE [Students] SET DATE_CORRELATION_OPTIMIZATION OFF
GO
ALTER DATABASE [Students] SET TRUSTWORTHY OFF
GO
ALTER DATABASE [Students] SET ALLOW_SNAPSHOT_ISOLATION OFF
GO
ALTER DATABASE [Students] SET PARAMETERIZATION SIMPLE
GO
ALTER DATABASE [Students] SET READ_COMMITTED_SNAPSHOT OFF
GO
ALTER DATABASE [Students] SET HONOR_BROKER_PRIORITY OFF
GO
ALTER DATABASE [Students] SET RECOVERY SIMPLE
GO
ALTER DATABASE [Students] SET MULTI_USER
GO
ALTER DATABASE [Students] SET PAGE_VERIFY CHECKSUM
GO
ALTER DATABASE [Students] SET DB_CHAINING OFF
GO
ALTER DATABASE [Students] SET FILESTREAM( NON_TRANSACTED_ACCESS = OFF )
GO
ALTER DATABASE [Students] SET TARGET_RECOVERY_TIME = 0 SECONDS
GO
USE [Students]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[StudentTable](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Name] [varchar](50) NULL,
[StudentClass] [varchar](50) NULL,
CONSTRAINT [PK_StudentTable] PRIMARY KEY CLUSTERED
(
[Id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
SET ANSI_PADDING OFF
GO
SET IDENTITY_INSERT [dbo].[StudentTable] ON
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass]) VALUES (1, N'Ramesh', N'X')
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass]) VALUES (2, N'Suersh', N'XI')
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass]) VALUES (3, N'Pretty', N'XII')
INSERT [dbo].[StudentTable] ([Id], [Name], [StudentClass]) VALUES (4, N'Dan', N'X')
SET IDENTITY_INSERT [dbo].[StudentTable] OFF
USE [master]
GO
ALTER DATABASE [Students] SET READ_WRITE
GO
Now create Entity framework(ADO.NET) file to connect the project with database, you would have to add Entity Data Model to your project by right-clicking the "Model" folder in your Solution Explorer and then click on Add and then New Item option of the Context Menu.
From the Add New Item window, select ADO.NET Entity Data Model and set its Name as "StudentModel"and then click Add.
Then the Entity Data Model Wizard will open up where you need to select EF Designer database option.
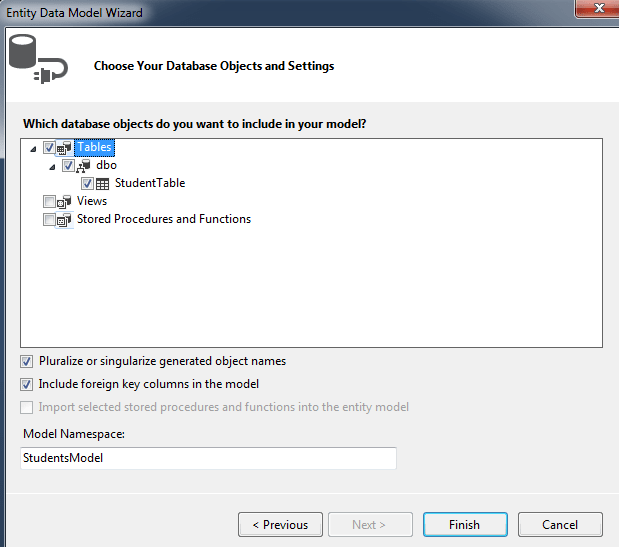
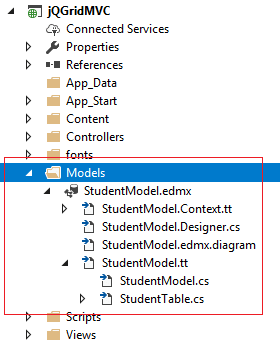
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace jQGridMVC.Controllers
{
public class JqgridController : Controller
{
// GET: Jqgrid
public ActionResult Index()
{
return View();
}
}
}
In the above index method, right click & select "Add View" and place the code as below
@{
ViewBag.Title = "jQGrid List";
}
<link href="~/Content/themes/base/jquery-ui.css" rel="stylesheet" />
<link href="~/Content/jquery.jqGrid/ui.jqgrid.css" rel="stylesheet" />
<h2>jQGrid List</h2>
<div>
<table id="grid"></table>
<div id="pager"></div>
</div>
@section scripts{
<script src="~/Scripts/jquery-ui-1.10.0.min.js"></script>
<script src="~/Scripts/i18n/grid.locale-en.js"></script>
<script src="~/Scripts/jquery.jqGrid.min.js"></script>
<script src="~/Scripts/script.js"></script>
}
In the above code please check if URL of scripts and CSS matches with your project URL for each file
Step 7: Add code in controller to send data to view as JSON, so now complete code of controller will be as below
using jQGridMVC.Models;
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace jQGridMVC.Controllers
{
public class JqgridController : Controller
{
// GET: Jqgrid
public ActionResult Index()
{
return View();
}
StudentsEntities db = new StudentsEntities();
public JsonResult GetValues(string sidx, string sord, int page, int rows) //Gets the todo Lists.
{
int pageIndex = Convert.ToInt32(page) - 1;
int pageSize = rows;
var Results = db.StudentTables.Select(
a => new
{
a.Id,
a.Name,
a.StudentClass,
});
int totalRecords = Results.Count();
var totalPages = (int)Math.Ceiling((float)totalRecords / (float)rows);
if (sord.ToUpper() == "DESC")
{
Results = Results.OrderByDescending(s => s.Id);
Results = Results.Skip(pageIndex * pageSize).Take(pageSize);
}
else
{
Results = Results.OrderBy(s => s.Id);
Results = Results.Skip(pageIndex * pageSize).Take(pageSize);
}
var jsonData = new
{
total = totalPages,
page,
records = totalRecords,
rows = Results
};
return Json(jsonData, JsonRequestBehavior.AllowGet);
}
// TODO:insert a new row to the grid logic here
[HttpPost]
public string Create([Bind(Exclude = "Id")] StudentTable obj)
{
string msg;
try
{
if (ModelState.IsValid)
{
db.StudentTables.Add(obj);
db.SaveChanges();
msg = "Saved Successfully";
}
else
{
msg = "Validation data not successfull";
}
}
catch (Exception ex)
{
msg = "Error occured:" + ex.Message;
}
return msg;
}
public string Edit(StudentTable obj)
{
string msg;
try
{
if (ModelState.IsValid)
{
db.Entry(obj).State = EntityState.Modified;
db.SaveChanges();
msg = "Saved Successfully";
}
else
{
msg = "Validation data not successfull";
}
}
catch (Exception ex)
{
msg = "Error occured:" + ex.Message;
}
return msg;
}
public string Delete(int Id)
{
StudentTable list = db.StudentTables.Find(Id);
db.StudentTables.Remove(list);
db.SaveChanges();
return "Deleted successfully";
}
}
}
In the above method, we are
- Using GetValues JSON method we are binding the db data to the jqgrid in the format of JSON (javascript object notation).
- Here parameters sidx means serial index; sort variable for sorting order; page numbers is for pages; and row parameter takes the numbers rows in jqgrid.
- Finally, we are binding data using JSON() method as a JSON object.
Step 8: Create jquery code for JQGrid to load data and perform CRUD operations as below
$(function()
{
debugger;
$("#grid").jqGrid
({
url: "/Jqgrid/GetValues",
datatype: 'json',
mtype: 'Get',
//table header name
colNames: ['Id', 'Name', 'StudentClass'],
//colModel takes the data from controller and binds to grid
colModel: [
{
key: true,
hidden: true,
name: 'Id',
index: 'Id',
editable: true
}, {
key: false,
name: 'Name',
index: 'Name',
editable: true
}, {
key: false,
name: 'StudentClass',
index: 'StudentClass',
editable: true
}
],
pager: jQuery('#pager'),
rowNum: 10,
rowList: [10, 20, 30, 40],
height: '100%',
viewrecords: true,
caption: 'Jq grid sample Application',
emptyrecords: 'No records to display',
jsonReader:
{
root: "rows",
page: "page",
total: "total",
records: "records",
repeatitems: false,
Id: "0"
},
autowidth: true,
multiselect: false
//pager-you have to choose here what icons should appear at the bottom
//like edit,create,delete icons
}).navGrid('#pager',
{
edit: true,
add: true,
del: true,
search: false,
refresh: true
}, {
// edit options
zIndex: 100,
url: '/Jqgrid/Edit',
closeOnEscape: true,
closeAfterEdit: true,
recreateForm: true,
afterComplete: function(response) {
if (response.responseText) {
alert(response.responseText);
}
}
}, {
// add options
zIndex: 100,
url: "/Jqgrid/Create",
closeOnEscape: true,
closeAfterAdd: true,
afterComplete: function(response) {
if (response.responseText) {
alert(response.responseText);
}
}
}, {
// delete options
zIndex: 100,
url: "/Jqgrid/Delete",
closeOnEscape: true,
closeAfterDelete: true,
recreateForm: true,
msg: "Are you sure you want to delete this student?",
afterComplete: function(response)
{
if (response.responseText)
{
alert(response.responseText);
}
}
});
});
Step 9: You are done with all the things build your project and run it in browser you will get data with output as below
Now try to Edit "Dan" row by selecting row and clicking edit
It will be updated in the database and in the table.
Try to add new entry by Clicking "+"(plus) icon
You will get updated table as below
Now test deleting the data by selecting/clicking a row and then you will get this dialog box
Click on "Delete", to remove the selected row(here it is 4th row of "Danny") & table will be updated.
Here is the updated SQL table after performing all the operations